Random names in C# using LINQ and a touch of functional programming
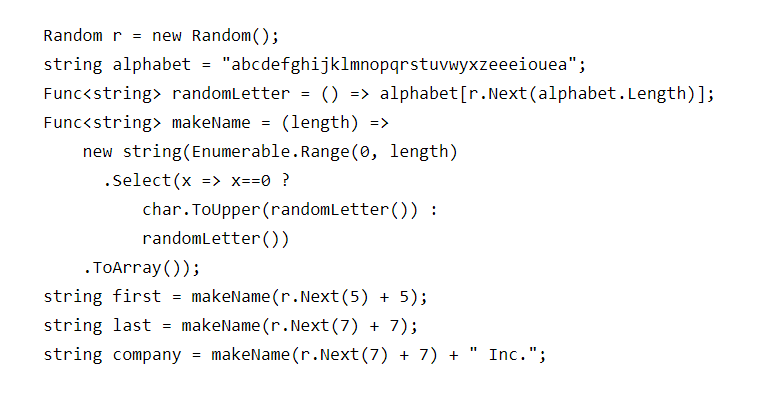
Today I needed some random names for testing. I wanted them to look like real names but to also look obviously made up so that nobody would ever treat them as a real record in the database. Below is a quick C# snippet to generate random names. It uses LINQ and a couple of anonymous functions to accomplish a task that would have taken many more lines in a traditional procedural style. Enumerable.Range() in particular is a really handy method for generating stuff.
Random r = new Random();
string alphabet = "abcdefghijklmnopqrstuvwyxzeeeiouea";
Func<string> randomLetter = () => alphabet[r.Next(alphabet.Length)];
Func<string> makeName = (length) =>
new string(Enumerable.Range(0, length)
.Select(x => x==0 ?
char.ToUpper(randomLetter()) :
randomLetter())
.ToArray());
string first = makeName(r.Next(5) + 5);
string last = makeName(r.Next(7) + 7);
string company = makeName(r.Next(7) + 7) + " Inc.";