My love/hate relationship with Stackoverflow
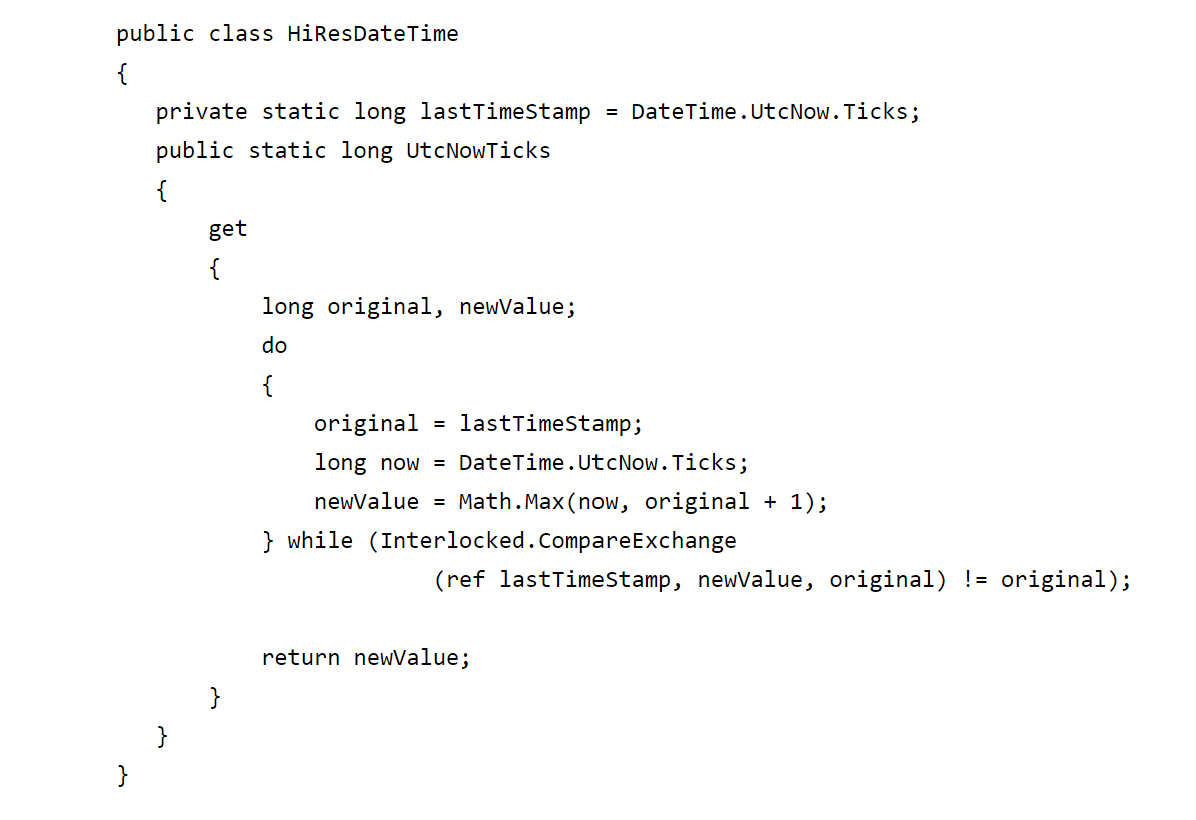
Stackoverflow is a great resource for any developer but sometimes it's a source of frustration. The rule that subjective questions get closed automatically is particularly annoying since it eliminates many interesting, expert-level responses that could have helped developers make smart technology decisions. Some of the earlier questions before this rule was enacted have great discussions around technology choices or framework comparisons. These days I mostly answer questions by commenting instead of posting answers because the bar for a comment is lower but there are a few answers I'm proud of.
These are not my top-rated answers by score because score has nothing to do with how hard the question was or how interesting the answer is; most of my highest rated answers are for trivial questions.
How to ensure a timestamp is always unique
I'd needed something like this myself in a project an wanted a fast, unique timestamp for recording against logs. Avoiding a lock makes this faster than other solutions and illustrates one way to use Interlocked.CompareExchange
:
public class HiResDateTime
{
private static long lastTimeStamp = DateTime.UtcNow.Ticks;
public static long UtcNowTicks
{
get
{
long original, newValue;
do
{
original = lastTimeStamp;
long now = DateTime.UtcNow.Ticks;
newValue = Math.Max(now, original + 1);
} while (Interlocked.CompareExchange
(ref lastTimeStamp, newValue, original) != original);
return newValue;
}
}
}
How to sort a database randomly
One way to achieve efficiently is to add a column to your data Shuffle that is populated with a random int (as each record is created). The query to access the table then becomes ...
Random random = new Random();
int seed = random.Next();
result = result.OrderBy(s => (~(s.Shuffle & seed)) & (s.Shuffle | seed));
// formula is equivalent to ^ (xor) seed but works when translated to SQL
This does an XOR operation in the database and orders by the results of that XOR.
Advantages:- Efficient: SQL handles the ordering, no need to fetch the whole table Repeatable: (good for testing) - can use the same random seed to generate the same random order Works on most (all?) Entity Framework supported databases
Where to store user uploads to a web-site
It's not as easy as it seems but I think I captured most of the rules that need to be observed to safely accept user uploads to a website.
You should NOT store the user uploads anywhere they can be directly accessed by a known URL within your site structure. This is a security risk as users could upload .htm file and .js files. Even a file with the correct extension can contain malicious code that can be executed in the context of your site by an authenticated user allowing server-side or client-side attacks.
See for example link and What security issues appear when users can upload their own files? which mention some of the issues you need to be aware of before you allow users to upload files and then present them for download within your site.
Don't put the files within your normal web site directory structure
Don't use the original file name the user gave you. You can add a content disposition header with the original file name so they can download it again as the same file name but the path and file name on the server shouldn't be something the user can influence.
Don't trust image files - resize them and offer only the resized version for subsequent download
Don't trust mime types or file extensions, open the file and manipulate it to make sure it's what it claims to be.
Limit the upload size and time.